Software Flow Chart
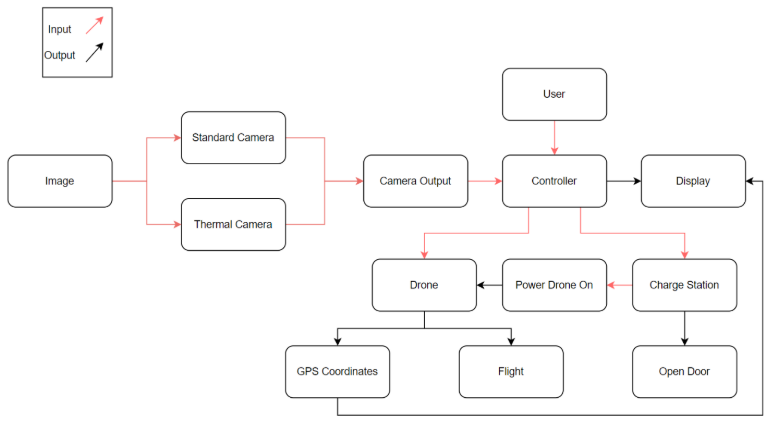
Thermal Camera Code
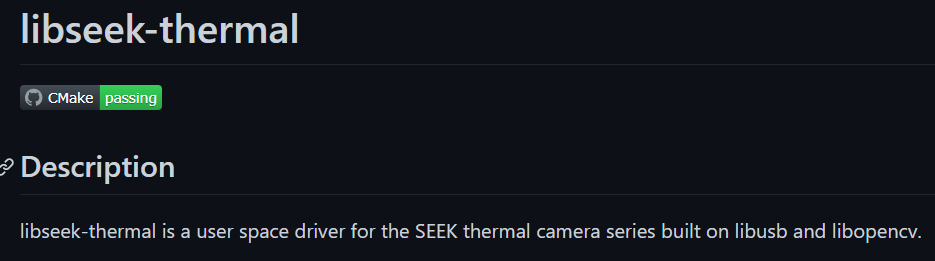
The code for the thermal camera to work with the raspberry pi was adapted from the open source library, libseek-thermal.
Door Motor Arduino Code
// Include the Arduino Stepper Library
#include <Stepper.h>
// Number of steps per output rotation
const int stepsPerRevolution = 200;
const int interruptPin = 21;
bool closed = true;
bool pressed = false;
// Create Instance of Stepper library
Stepper myStepper(stepsPerRevolution, 2, 3, 5, 4);
void setup()
{
// set the speed at 60 rpm:
myStepper.setSpeed(60);
// initialize the serial port:
Serial.begin(9600);
attachInterrupt(digitalPinToInterrupt(interruptPin), spinMotor, RISING);
}
void loop()
{
if (closed && pressed){
myStepper.step(stepsPerRevolution * 3);
delay(500);
pressed = false;
}
if (!(closed) && pressed){
myStepper.step(-stepsPerRevolution * 3);
delay(500);
pressed = false;
}
}
void spinMotor(){
pressed = true;
if (closed){
closed = false;
} else {
closed = true;
}
}